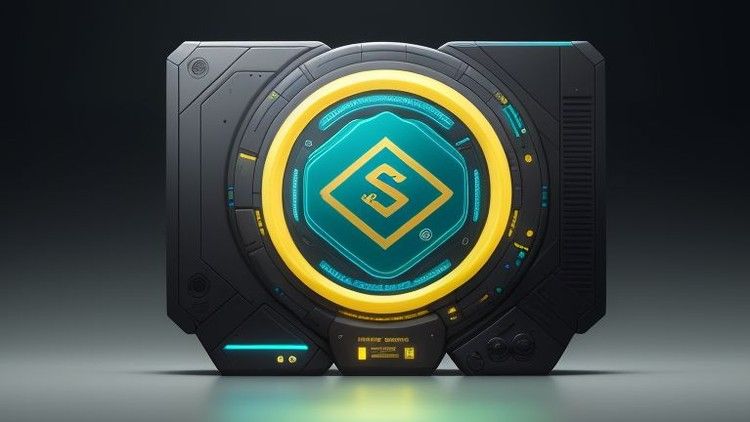
Advanced Skill Test: Python Professional Level 1 (PCPP1™)
Description
The PCPP1™ certification is one of the two professional-level certifications offered by the Python Institute. It focuses on testing candidates' knowledge of Python at an advanced level, covering topics such as Object-Oriented Programming (OOP), file processing, multithreading, database interactions, and more. This course will guide you through the essential concepts required to pass the PCPP1™ exam and to write professional-grade Python code in real-world scenarios.
Course Structure
The course is structured into modules that progressively build on each other. Starting with the fundamentals of advanced Python, you will explore in-depth topics such as OOP, threading, multiprocessing, and testing, followed by hands-on projects and coding exercises. Each module is designed to provide a balanced combination of theory and practical coding experience, ensuring you fully grasp advanced Python concepts.
What You Will Learn
- Advanced Object-Oriented Programming (OOP)
Object-Oriented Programming is a core pillar of Python, and mastering OOP is essential for writing maintainable and scalable code. In this course, you'll learn to:- Implement advanced OOP concepts, such as inheritance, polymorphism, and encapsulation.
- Use abstract base classes (ABC) and multiple inheritance.
- Understand metaclasses and how to create custom class behaviors.
- Overload operators and implement magic methods like __init__, __str__, and __repr__.
- GUI Programming with Tkinter
Graphical User Interfaces (GUIs) make applications user-friendly and interactive. You'll learn to use Tkinter, Python’s standard GUI library, to:- Build user interfaces with buttons, labels, textboxes, and more.
- Handle events such as mouse clicks and key presses.
- Customize the appearance of your GUI applications with themes and styles.
- Create dialog boxes, windows, and menus for more interactive experiences.
- File Processing and Data Manipulation
Reading and processing data from files is a fundamental skill for any Python developer. This module will teach you:- How to read and write files in different formats (CSV, JSON, XML).
- How to parse structured data and handle large data sets efficiently.
- The use of context managers (with statements) for safe file handling.
- Techniques for handling binary data and working with file streams.
- Multithreading and Multiprocessing
For high-performance applications, it's often necessary to run multiple tasks simultaneously. You'll explore:- The basics of multithreading and how to manage threads in Python.
- How to synchronize threads and handle race conditions with locks and semaphores.
- The multiprocessing module to take full advantage of multiple CPU cores.
- The use of queues and pipes for communication between processes.
- Testing and Test-Driven Development (TDD)
Testing is essential for ensuring that your code works as expected. In this course, you will:- Learn how to write effective unit tests using Python’s unittest framework.
- Explore test-driven development (TDD) techniques to write tests before code.
- Use the pytest module for more advanced testing features, such as parameterized tests and fixtures.
- Learn how to mock objects and external services to isolate your tests.
- Best Practices for Writing Clean, Efficient Python Code
Writing maintainable and efficient code is a key aspect of professional programming. You will learn:- Python’s PEP 8 coding conventions for readability and consistency.
- How to profile your code and optimize performance.
- Common design patterns like Singleton, Factory, and Observer and how to implement them in Python.
- How to write clean, readable code using meaningful variable names, comments, and docstrings.
- Working with Databases
Data storage is an essential part of most applications. This course will teach you:- How to connect to relational databases using Python’s sqlite3 module.
- How to interact with databases, perform SQL queries, and handle results.
- Basics of working with NoSQL databases, such as MongoDB, for scalable data storage.
- Advanced Python Libraries and Modules
Python's standard library is vast and powerful, and in this course, you’ll explore advanced modules like:- Regular Expressions (re) for pattern matching and text processing.
- Datetime for handling date and time-related data and working with time zones.
- Socket Programming for creating networking applications and handling protocols.